Pointers in C++ not to be scared of!
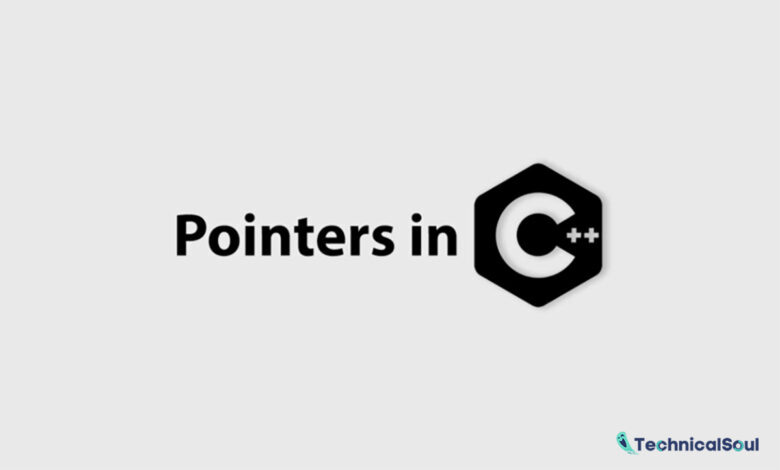
What are pointers in C++ programming language?
Pointers in C++ are a nightmare to newbie programmers, many aspiring C++ learners have gotten stuck on them. We’ll see shortly that they aren’t to be scared of.
Consider a normal variable, like the one below:
int normal_variable{ 102 };
Let’s say that the integer variable normal_variable is assigned memory address 110234. As we know that we use variable names to refer to the data we have stored in memory somewhere. Whenever we use the name normal_variable in an expression or statement, we’re accessing the data i.e. 102 stored at memory location 110234. Take a look at the figure below:
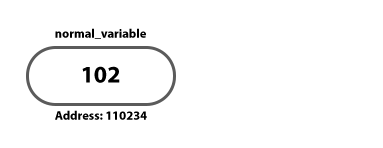
The nice thing about variables is that we don’t have to worry about the each memory address, we only use the variable name to refer to the address, compiler translates all the variables into appropriate memory address at compilation time. Now comes the pointer.
Pointers in C++
Pointers in C++ are variables used to hold the addresses of another variables as their values. The only reason to store the addresses of another variables is to use them later whenever required.
To declare a pointer, we simply define a variable with a pointer type as you can see below:
int* pointer_variable;
pointer_variable = &normal_variable;
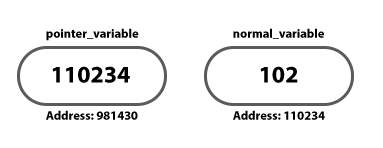
Visually, you can see the concept of a pointer. Variable pointer_variable holds the address of normal_variable, the address can be availed by using the operator&, that returns the address of a variable. However, why use the pointer_variable to hold the address of normal_variable if we can directly refer to the normal_variable by name?
The answer is simple. You can refer to a named variable in the source code directly but what if you want to refer to a variable which doesn’t have a name? There the pointer helps, you use pointer to store the address for later use.
Heap memory and pointers in C++
The RAM is generally divided into many sections. Two of those sections are Stack memory and Heap memory. Stack memory is also called automatic memory and Heap is also called dynamic memory.
Automatic memory: Memory allocation and de-allocation is managed by the C++.
Dynamic memory: Memory allocation and de-allocation is managed by you as the programmer.
Need for Dynamic Memory Allocation
When the program needs additional memory at the running time, it can use the Heap memory. A programmer is responsible for allocating and de-allocating the dynamic memory.
The only way around using the dynamic memory is with the help of pointers. There is no other way possible in C++.
Heap memory can only be accessed using pointers. Pointers store the address of memory allocated by the programmers. Here’s a sample program and the visual representation below for better understanding of pointers:
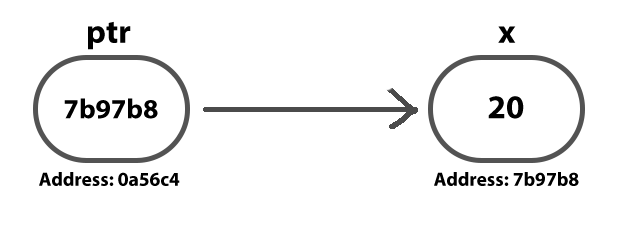
#include <iostream>
int main()
{
// variable declaration
int x{ 20 };
// int type pointer declaration and initialization
// ptr storing the address of variable x
int* ptr{ &x };
// Note: '*' is a de-reference operator to access the data through pointer
std::cout << *ptr << '\n';
return 0;
}
Output: 20
Note: The example above doesn’t allocate memory dynamically, the pointer is pointing to the stack memory variable x. Remember, no memory is allocated dynamically on the heap here, so need to de-allocate the memory. De-allocation is usually done using the delete operator followed by the pointer name.
Conclusion
As I said in the beginning, the pointers aren’t to be scared of.
Always remember, dynamic memory has a dynamic duration. The memory will stay allocated until you de-allocate it or the program terminates. Be careful when using the pointers. The most common mistake programmers make when they forget to delete the dynamic memory used by the program.
Check out my another post on why learn C++!